
Introduction to containerization and Cloud Run
Containerization is a technique that packages applications and their dependencies into isolated environments called containers. These containers offer consistency across various environments, ensuring that applications run reliably regardless of the underlying infrastructure.
Docker, a leading containerization platform, introduced the concept of Docker images, which serve as the building blocks for containers.
Docker images are snapshots of a specific file system, along with the application code, runtime, libraries, and settings required to run the application. These images are created from a Dockerfile - a text file that outlines the instructions for building the image. By encapsulating everything needed for an application to run, Docker images eliminate compatibility issues and create a standardized environment.
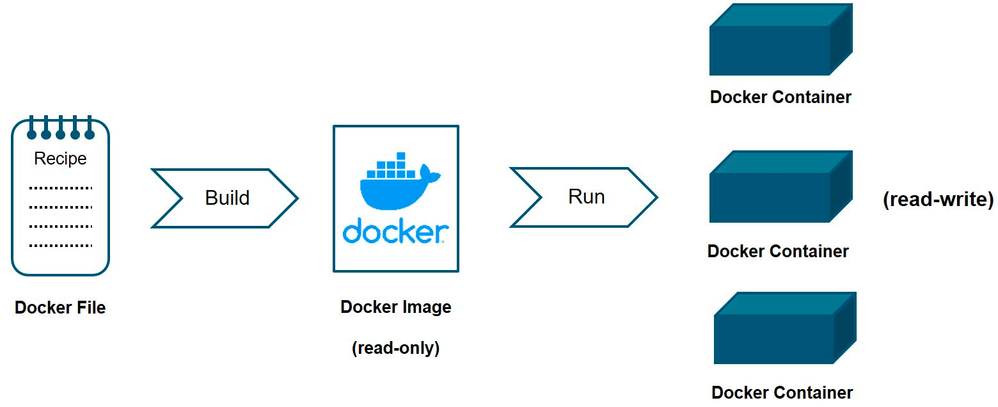
Cloud Run is a serverless compute platform by Google that brings containerization and serverless concepts together. It allows developers to deploy containerized applications without managing the underlying infrastructure. Cloud Run abstracts away the complexities of scaling, load balancing, and resource provisioning, enabling developers to focus solely on the application code.
Cloud Run is a fully managed service, so you don't have to worry about managing the underlying infrastructure. You simply deploy your containerized application to Cloud Run and Cloud Run will take care of the rest.
Cloud Run is highly scalable and can handle any amount of traffic. Cloud Run also provides a number of features that make it easy to develop, deploy, and manage your applications, such as:
- Automatic scaling: Cloud Run automatically scales your application up and down based on traffic.
- Zero-configuration networking: Cloud Run provides a secure and reliable network connection for your application.
- Automatic deployment: Cloud Run automatically deploys your application when you push a new container image to Cloud Build.
- Integrated logging and monitoring: Cloud Run provides integrated logging and monitoring for your application.

Cloud Run memory leaks: Deep dive into out-of-memory issues for Spring Boot applications
Cloud Run
Cloud Run simplifies deploying and scaling containerized applications (like Spring Boot apps) without infrastructure management. It offers two CPU allocation options:
- Automatic: Resources dynamically scale up and down based on actual usage, making it cost-effective for fluctuating workloads.
- Always allocated: A minimum number of CPUs are always reserved, offering faster cold starts and predictable performance, but incurring constant cost.

Understanding memory leaks in Spring Boot applications
Memory leaks occur when allocated memory isn't released even after it's no longer needed, leading to performance issues and crashes. Here's a closer look at the causes:
1. Unreleased objects and resources:
- Holding references to objects beyond their required lifespan prevents garbage collection and causes memory bloat.
- Ensure proper closure of connections, streams, and other resources after use.
- Review code for unnecessary object creation and implement efficient release mechanisms.

2. Inefficient data structures
- Using inefficient data structures like large lists or arrays can consume excessive memory.
- For large datasets, utilize optimized structures like Sets or Hashes for efficient storage and access.
- Analyze data structure choices and optimize them based on data characteristics and access patterns.

3. Excessive caching practices
- Overly aggressive caching without eviction policies can lead to memory exhaustion.
- Implement efficient caching mechanisms like LRU (Least Recently Used) or TTL (Time To Live) to automatically discard unused data.
- Define appropriate cache size limits and eviction strategies based on application requirements.

4. Unclosed database connections
- Leaking database connections, especially in connection pools, can quickly consume available memory.
- Ensure proper connection closing and release, using efficient connection pool management techniques.
- Implement connection validation and timeout mechanisms to prevent orphaned connections.
5. Third-party library issues
- External libraries like JPA or Hibernate might have internal memory leaks or inefficient resource handling.
- Choose well-maintained libraries with efficient memory management and update them regularly.
- Monitor library usage and analyze their resource consumption patterns to identify potential leaks.
6. Redis connection
- Unreleased Redis resources: Failing to close Redis connections and releasing resources like connections and result sets can lead to memory leaks.
- Object accumulation from Redis data: Accumulating data retrieved from Redis in memory without proper object release can cause memory exhaustion.
- Inefficient data structures for Redis data: Using inefficient data structures to store data retrieved from Redis can unnecessarily consume memory.
- Excessive caching of Redis data: Caching large amounts of data from Redis without eviction policies can lead to memory exhaustion.
- Unoptimized libraries for Redis interaction: Third-party libraries used for Redis interaction might have memory leaks due to inefficient resource management.
Identifying and fixing memory leaks
- Monitoring: Use Cloud Monitoring to track memory usage over time, identifying trends and spikes for potential leaks.
- Profiling tools: Utilize tools like Cloud Profiler and Heapster to analyze memory allocation and object creation patterns.
- Code review: Thoroughly review your code, focusing on resource handling, data structures, and library usage to identify leaks.
- Garbage collector tuning: Optimize garbage collector settings for efficient object cleanup and memory management.
- Load testing: Conduct simulated high traffic scenarios to observe memory behavior and proactively detect leaks.
Preventative measures for memory leaks
- Coding best practices: Follow best practices such as resource management, efficient data structures, and object lifecycle handling.
- Optimized libraries: Choose libraries known for efficient resource utilization and update them regularly.
- Efficient caching: Implement caching strategies with eviction policies to prevent memory overload.
- Monitoring and analysis: Regularly monitor memory usage and analyze trends to identify potential issues before they escalate.
Conclusion
By understanding the various causes of memory leaks, utilizing tools and techniques for identification and fixing, and implementing preventative measures, you can ensure your Spring Boot applications run smoothly and efficiently on Cloud Run, minimizing out-of-memory issues and promoting reliable performance.
Authored by:
- Utkarsh Bhardwaj (@bhardwaju) Senior Solutions Consultant, Google
- Abhishek Patil (@psabhishek), Cloud Migration Consultant, Google
- Komal Bisht (@bishtkomal), Cloud Migration Consultant, Google